2023. 6. 22. 16:17ใCoding Test/Programmers
๐๋ฌธ์ ๋ณด๋ฌ๊ฐ๊ธฐ
ํ๋ก๊ทธ๋๋จธ์ค
์ฝ๋ ์ค์ฌ์ ๊ฐ๋ฐ์ ์ฑ์ฉ. ์คํ ๊ธฐ๋ฐ์ ํฌ์ง์ ๋งค์นญ. ํ๋ก๊ทธ๋๋จธ์ค์ ๊ฐ๋ฐ์ ๋ง์ถคํ ํ๋กํ์ ๋ฑ๋กํ๊ณ , ๋์ ๊ธฐ์ ๊ถํฉ์ด ์ ๋ง๋ ๊ธฐ์ ๋ค์ ๋งค์นญ ๋ฐ์ผ์ธ์.
programmers.co.kr
๐จโ๐ปํ์ด ๊ณผ์
์
๋ ฅ์ผ๋ก ์ฃผ์ด์ง๋ ๋ฐฐ์ด ํฌ๊ธฐ๊ฐ ์ต๋
- ๋ (land)์ ์ฒซ ํ์ dp์ ๊ทธ๋๋ก ์ ์ฅํด๋๋ค.
- ํ์ฌ ํ๊ณผ ์ด์ row, col์ด๋ผ๊ณ ํ ๋, dp[row - 1]์์ col์ ์ ์ธํ ์ต๋๊ฐ์ ์ฐพ์ newDpRow์ ์ ์ฅํ๋ค.
- dp์ newDpRow๋ฅผ ์ถ๊ฐํ๋ค.
- 2, 3๋ฒ ๊ณผ์ ์ land์ ๋ง์ง๋ง ํ๊น์ง ๋ฐ๋ณตํ๋ค.
- ๋ค ๋ฐ๋ณตํ๋ค๋ฉด, dp์ค์์ ์ต๋๊ฐ์ ๋ฆฌํดํ๋ค.
๋์ ํ๋ก๊ทธ๋๋ฐ์ ๋ง์ด ์ฌ์ฉํด ๋ณธ ์ ์ด ์์ด์, ์ฝ๋๋ฅผ ์์ฑํด๋ณด๋ ๋ฐ์ ๊ฝค๋ ์ ๋จน์์ต๋๋ค. DP ๋ฌธ์ ๋ ์ด์ ์ข ๋ง์ด ํ์ด๋ด์ผ๊ฒ ๋ค์. ์๋๋ ์ ์์ค ์ฝ๋์ ๋๋ค.
โ๏ธ์์ค ์ฝ๋ ๋ฐ ๊ฒฐ๊ณผ
#include <vector>
#include <algorithm>
using namespace std;
int GetMaxValue(const vector<int>& row, int exceptColumn) {
int maxValue = 0;
for (int i = 0; i < row.size(); i++) {
if (exceptColumn == i)
continue;
maxValue = max(maxValue, row[i]);
}
return maxValue;
}
int solution(vector<vector<int>> land)
{
vector<vector<int>> dp(1, vector<int>(land[0].begin(), land[0].end()));
for (int row = 1; row < land.size(); row++) {
vector<int> dpRow;
for (int col = 0; col < land[row].size(); col++) {
int preDpRowMaxValue = GetMaxValue(dp[row - 1], col);
dpRow.emplace_back(preDpRowMaxValue + land[row][col]);
}
dp.emplace_back(dpRow);
}
int lastRow = dp.size() - 1;
return *max_element(dp[lastRow].begin(), dp[lastRow].end());
}
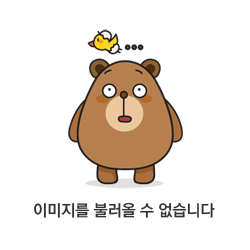
๋ค๋ฅธ ๋ถ์ ๋ชจ๋ฒ ์ฝ๋ ํ์ต
#include <vector>
#include <algorithm>
using namespace std;
int solution(vector<vector<int> > land)
{
for(int i = 0; i < land.size() - 1; i++)
{
land[i + 1][0] += max(land[i][1], max(land[i][2], land[i][3]));
land[i + 1][1] += max(land[i][0], max(land[i][2], land[i][3]));
land[i + 1][2] += max(land[i][1], max(land[i][0], land[i][3]));
land[i + 1][3] += max(land[i][1], max(land[i][2], land[i][0]));
}
return *max_element(land[land.size() - 1].begin(), land[land.size() - 1].end());
}
- max(), min() ์ด๋ฐ ํจ์๋ค์ ์ธ์๋ฅผ 2๊ฐ๋ง ๋ฐ์ ์ ์๋ ์ค ์์๋๋ฐ, max({,1, 2, 3}) ์ด๋ฐ ์์ผ๋ก { }๋ฅผ ํตํด ์ธ์๋ฅผ ์ฃผ๊ฒ ๋๋ฉด ๋ฐฐ์ด๋ก ๋ฐ์์ ๋์ํ๋ ๋ด ๋๋ค. ๋์ค์ ์ ์ฉํ๊ฒ ์ธ ์ ์์ ๊ฒ ๊ฐ์์.
- ๋ (land)์ ์ด์ ํ์ ๋ณผ ํ์๊ฐ ์์ผ๋ฏ๋ก, ๋ค์ ํ์๋ค๊ฐ ํ์ฌ ํ์ ์ต๋๊ฐ์ ๋ํด์ ์ ์ฅํด๋๋ค์. ๋ฉ๋ชจ๋ฆฌ๋ฅผ ๋ณ๋๋ก ์ ์จ๋ ๋๋ ๊ต์ฅํ ์ข์ ๊ฒ ๊ฐ์ต๋๋ค.
'Coding Test > Programmers' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[Programmers] Lv2. ๊ฒ์ ๋งต ์ต๋จ๊ฑฐ๋ฆฌ | C++ (0) | 2023.06.22 |
---|---|
[Programmers] Lv2. ์ฃผ์๊ฐ๊ฒฉ | C++ (0) | 2023.06.22 |
[Programmers] Lv2. ์คํ์ฑํ ๋ฐฉ | C++ (0) | 2023.06.21 |
[Programmers] Lv2. [3์ฐจ] n์ง์ ๊ฒ์ | C++ (0) | 2023.06.21 |
[Programmers] Lv2. ๋ ๋งต๊ฒ | C++ (0) | 2023.06.21 |